Top Services
Our Working Process in 3 Steps
Adipiscing elit, sed do euismod tempor incidunt ut labore et dolore magna aliqua. Ut enim ad minim.
Discovery
Natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam.
Design & Refine
Natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam.
Implementation
Natus error sit voluptatem accusantium doloremque laudantium, totam rem aperiam.
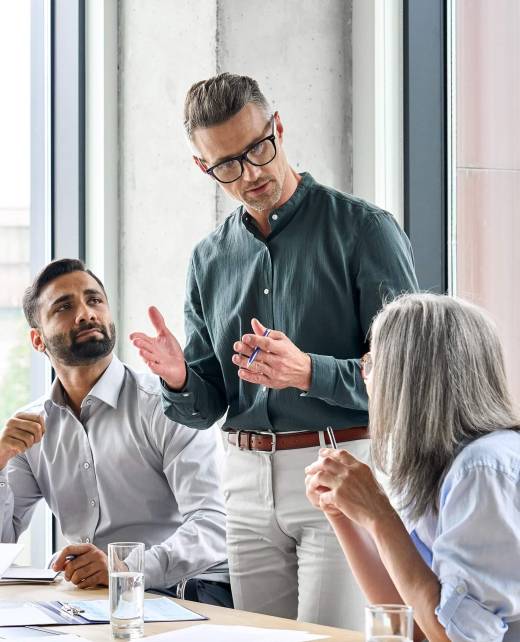
We build
better products
stage 1
Client's Brief
Architecto beatae vitae dicta sunt explicabo. Nemo enim ipsam voluptatem lorem ipsum dol. Unde omnis iste natus error sit voluptatem accusantium dolorem que laudantium, totam rem ap. Adipiscing elit, sed do euismod tempor incidunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitaci.
stage 2
Planning & Design
Dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aut odit aut fugit, sed quia consequuntur magni.
- Technical Discovery
- Technical Architecture
- Customer Experience
- Effective Refine
- Constructive Ideas
- Digital Gadgets
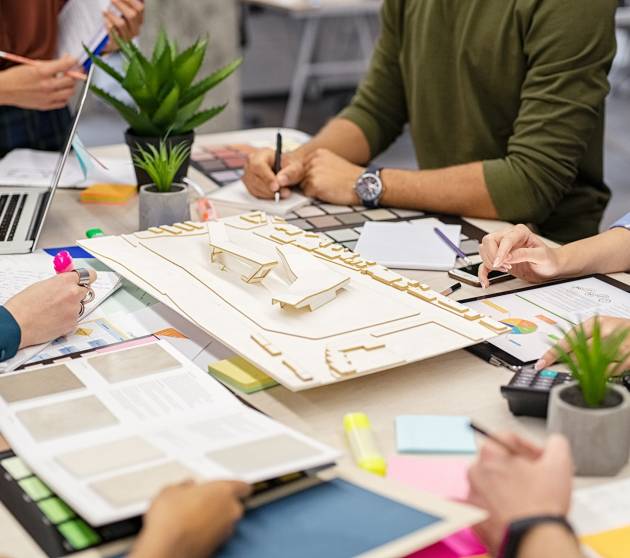
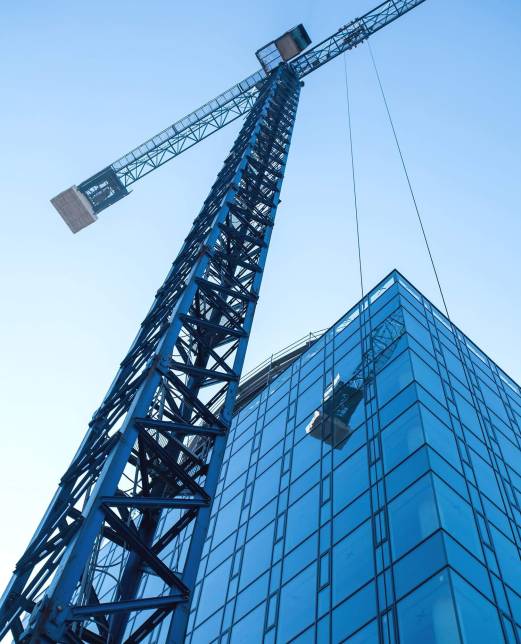
stage 3
Project Fulfillment
Dicta sunt explicabo. Nemo enim ipsam voluptatem quia voluptas sit aspernatur aut odit aut fugit.
- Adipiscing elit sed euismod
- Tempor incidunt
- Labore et dolore magna
Contact Us
Have questions? Get in touch!
Adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim.